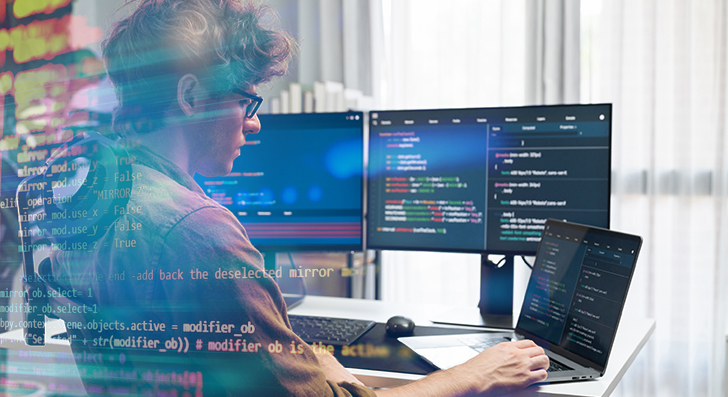
Scalability usually means your application can deal with advancement—additional customers, more details, plus more website traffic—with no breaking. As being a developer, setting up with scalability in your mind saves time and tension afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't a thing you bolt on later—it ought to be component of your respective strategy from the start. Numerous purposes fall short when they increase fast mainly because the original layout can’t handle the extra load. To be a developer, you should Believe early regarding how your system will behave under pressure.
Start out by creating your architecture to get adaptable. Stay away from monolithic codebases wherever almost everything is tightly related. Rather, use modular style and design or microservices. These styles break your app into scaled-down, unbiased components. Every single module or services can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from working day 1. Will it want to take care of a million consumers or maybe 100? Pick the ideal type—relational or NoSQL—depending on how your knowledge will improve. Strategy for sharding, indexing, and backups early, Even though you don’t need to have them nevertheless.
A further vital point is to avoid hardcoding assumptions. Don’t create code that only operates beneath present-day disorders. Think about what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design patterns that assist scaling, like concept queues or celebration-pushed programs. These support your app manage far more requests with out obtaining overloaded.
Whenever you Develop with scalability in mind, you are not just planning for achievement—you are lowering potential head aches. A very well-prepared technique is simpler to keep up, adapt, and expand. It’s far better to organize early than to rebuild later.
Use the Right Databases
Deciding on the appropriate database is really a key Element of making scalable programs. Not all databases are constructed the same, and utilizing the Mistaken you can slow you down or simply induce failures as your application grows.
Commence by understanding your facts. Could it be highly structured, like rows inside of a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. They are potent with associations, transactions, and regularity. Additionally they aid scaling tactics like read through replicas, indexing, and partitioning to handle far more visitors and data.
When your data is much more adaptable—like user exercise logs, item catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and can scale horizontally a lot more conveniently.
Also, think about your examine and compose styles. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be managing a hefty publish load? Take a look at databases that may tackle higher compose throughput, or maybe party-based info storage devices like Apache Kafka (for non permanent data streams).
It’s also intelligent to Consider in advance. You may not require Superior scaling characteristics now, but deciding on a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based upon your obtain styles. And always monitor database performance as you grow.
In short, the best database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve many issues later on.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start off by composing clean, very simple code. Prevent repeating logic and remove everything needless. Don’t choose the most advanced Resolution if a simple a person performs. Keep your capabilities quick, focused, and simple to test. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or makes use of far too much memory.
Following, take a look at your databases queries. These frequently gradual factors down more than the code by itself. Make sure Just about every query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and alternatively find precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who discover the exact same data currently being asked for over and over, use caching. Retail outlet the results briefly working with applications like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in teams. This cuts down on overhead and will make your application much more effective.
Make sure to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to handle one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it's to manage far more people plus much more targeted visitors. If all the things goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the operate, the load balancer routes consumers to various servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused rapidly. When consumers ask for the exact same more info details again—like an item web page or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two frequent sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers databases load, improves speed, and can make your application far more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they help your app cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to buy hardware or guess future capacity. When visitors raises, you'll be able to incorporate far more methods with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection applications. You may center on making your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This can make it quick to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular Software for this.
Whenever your app uses various containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy very easily, and Get better swiftly when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease chance, and help you remain centered on building, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your application is carrying out, place difficulties early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are doing. Resources like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this facts.
Don’t just watch your servers—observe your application too. Keep an eye on how long it takes for customers to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential troubles. By way of example, When your reaction time goes previously mentioned a limit or perhaps a services goes down, you should get notified immediately. This allows you take care of difficulties rapid, typically just before consumers even discover.
Checking is likewise valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you are able to roll it again ahead of it triggers real destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate equipment set up, you keep on top of things.
In a nutshell, monitoring will help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about understanding your process and making sure it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications require a solid foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Feel large, and Create good.